Querying Custom Metadata with GraphQL in LWC, allows developers to create reusable configuration data that can be packaged and deployed across different environments. Integrating Custom Metadata with GraphQL in Lightning Web Components (LWC) enhances your ability to fetch and manipulate this configuration data efficiently, leveraging the power of GraphQL’s flexible querying capabilities.
Understanding Custom Metadata
Custom Metadata Types are similar to custom objects but are primarily used for application configurations. They enable you to define metadata records that can be referenced in your Apex code, validation rules, and more, without hardcoding values. This makes your applications more scalable and maintainable.
Why Use GraphQL with Custom Metadata in LWC?
GraphQL provides a streamlined way to query only the data you need, reducing the amount of data transferred and improving performance. When combined with LWC, it allows for dynamic and efficient data retrieval, especially beneficial for configurations stored in Custom Metadata.
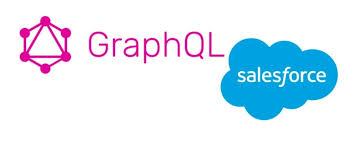
Steps to Query Custom Metadata with GraphQL in LWC
- Set Up a GraphQL Server:
- Since Salesforce doesn’t natively support GraphQL, you need to set up an external GraphQL server. You can use frameworks like Apollo Server or Express-GraphQL.
- Create resolvers that interact with Salesforce’s Custom Metadata using Salesforce’s REST API or Apex endpoints.
- Define Your GraphQL Schema:
- Define the types and queries that represent your Custom Metadata.
type CustomMetadata {
DeveloperName: String
MasterLabel: String
Field1: String
Field2: String
}
type Query {
getCustomMetadata: [CustomMetadata]
}
- Integrate GraphQL in LWC:
- Use the
fetch
API or a GraphQL client like Apollo to send queries from your LWC. - Handle authentication securely, typically using OAuth tokens.
- Example LWC JavaScript:
import { LightningElement, track } from 'lwc';
export default class CustomMetadataFetcher extends LightningElement {
@track metadata;
@track error;
connectedCallback() {
this.fetchCustomMetadata();
}
fetchCustomMetadata() {
const query = `
query {
getCustomMetadata {
DeveloperName
MasterLabel
Field1
Field2
}
}
`;
fetch('https://your-graphql-server.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${yourAccessToken}`
},
body: JSON.stringify({ query })
})
.then(response => response.json())
.then(data => {
this.metadata = data.data.getCustomMetadata;
})
.catch(error => {
this.error = error;
console.error('Error fetching Custom Metadata:', error);
});
}
}
- Display Data in LWC HTML:
<template>
<template if:true={metadata}>
<ul>
<template for:each={metadata} for:item="item">
<li key={item.DeveloperName}>
{item.MasterLabel}: {item.Field1}, {item.Field2}
</li>
</template>
</ul>
</template>
<template if:true={error}>
<p>Error: {error}</p>
</template>
</template>
Best Practices
- Secure Authentication: Always protect your GraphQL endpoints with proper authentication mechanisms.
- Optimize Queries: Only request the necessary fields to enhance performance.
- Error Handling: Implement robust error handling to manage failed queries gracefully.
- Caching: Use caching strategies to minimize redundant data fetching and improve load times.
Example: Querying Custom Metadata with GraphQL in Lightning Web Components (LWC)
In this example, we’ll demonstrate how to query Salesforce Custom Metadata using GraphQL within a Lightning Web Component (LWC). We’ll walk through defining a Custom Metadata Type, setting up a GraphQL server, and integrating the GraphQL query into an LWC to display the metadata in a user-friendly table.
Scenario Overview
Suppose you have a Custom Metadata Type named App_Config__mdt
that stores configuration settings for your Salesforce application. This metadata includes fields such as DeveloperName
, MasterLabel
, ApiEndpoint__c
, and EnableFeatureX__c
. We’ll create an LWC that fetches and displays this configuration data using GraphQL.
Step 1: Define Custom Metadata Type and Sample Records
Custom Metadata Type: App_Config__mdt
Field API Name | Field Label | Data Type |
---|---|---|
DeveloperName | Developer Name | Text |
MasterLabel | Master Label | Text |
ApiEndpoint__c | API Endpoint | URL |
EnableFeatureX__c | Enable Feature X | Checkbox |
Sample Custom Metadata Records
DeveloperName | MasterLabel | ApiEndpoint__c | EnableFeatureX__c |
---|---|---|---|
ConfigPrimary | Primary Configuration | https://api.primary.com/graphql | true |
ConfigSecondary | Secondary Configuration | https://api.secondary.com/graphql | false |
Step 2: Set Up a GraphQL Server
Since Salesforce doesn’t natively support GraphQL, you’ll need to set up an external GraphQL server. Here’s a high-level overview:
- Choose a Framework: Use Apollo Server or Express-GraphQL.
- Connect to Salesforce: Utilize Salesforce’s REST API or Apex endpoints to access Custom Metadata.
- Define Schema and Resolvers:
type AppConfig {
DeveloperName: String
MasterLabel: String
ApiEndpoint__c: String
EnableFeatureX__c: Boolean
}
type Query {
getAppConfigs: [AppConfig]
}
const resolvers = {
Query: {
getAppConfigs: async () => {
// Fetch Custom Metadata from Salesforce
// Return as an array of AppConfig objects
},
},
};
Ensure your GraphQL server is accessible and properly secured with authentication mechanisms like OAuth.
Step 3: Create the Lightning Web Component
3.1. LWC JavaScript File: appConfigTable.js
import { LightningElement, track } from 'lwc';
export default class AppConfigTable extends LightningElement {
@track appConfigs = [];
@track error;
// Define table columns
columns = [
{ label: 'Developer Name', fieldName: 'DeveloperName' },
{ label: 'Master Label', fieldName: 'MasterLabel' },
{ label: 'API Endpoint', fieldName: 'ApiEndpoint__c', type: 'url' },
{ label: 'Enable Feature X', fieldName: 'EnableFeatureX__c', type: 'boolean' }
];
// Fetch Custom Metadata on component load
connectedCallback() {
this.fetchAppConfigs();
}
// Method to fetch data from GraphQL server
fetchAppConfigs() {
const query = `
query {
getAppConfigs {
DeveloperName
MasterLabel
ApiEndpoint__c
EnableFeatureX__c
}
}
`;
fetch('https://your-graphql-server.com/graphql', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer YOUR_ACCESS_TOKEN` // Replace with your access token
},
body: JSON.stringify({ query })
})
.then(response => response.json())
.then(data => {
if (data.errors) {
throw new Error(data.errors[0].message);
}
this.appConfigs = data.data.getAppConfigs;
})
.catch(error => {
this.error = error.message;
console.error('Error fetching App Configurations:', error);
});
}
}
3.2. LWC HTML Template: appConfigTable.html
<template>
<lightning-card title="Application Configurations">
<template if:true={appConfigs}>
<lightning-datatable
key-field="DeveloperName"
data={appConfigs}
columns={columns}
hide-checkbox-column="true">
</lightning-datatable>
</template>
<template if:true={error}>
<div class="slds-text-color_error">
<p>Error: {error}</p>
</div>
</template>
</lightning-card>
</template>
3.3. LWC CSS File: appConfigTable.css
(Optional)
/* Optional styling for better presentation */
.slds-text-color_error {
padding: 1rem;
}
Step 4: Explanation of the Example
- Custom Metadata Setup:
- We defined a Custom Metadata Type
App_Config__mdt
with relevant fields. - Created sample records to represent different configurations.
- GraphQL Server Configuration:
- Set up a GraphQL server with a schema that includes a
getAppConfigs
query. - Implemented resolvers to fetch data from Salesforce’s Custom Metadata.
- Lightning Web Component Implementation:
- JavaScript (
appConfigTable.js
):- Imported necessary modules and defined tracked properties
appConfigs
anderror
. - Defined table columns corresponding to Custom Metadata fields.
- In the
connectedCallback
, invokedfetchAppConfigs
to retrieve data when the component loads. - The
fetchAppConfigs
method constructs a GraphQL query, sends a POST request to the GraphQL server, and processes the response. - Handles errors by setting the
error
property and logging to the console.
- Imported necessary modules and defined tracked properties
- HTML (
appConfigTable.html
):- Utilized
lightning-card
to create a styled container. - Used
lightning-datatable
to display the fetched Custom Metadata in a table format. - Included conditional rendering to display errors if any occur during data fetching.
- Utilized
- Authentication and Security:
- Ensured the GraphQL server endpoint is secured with proper authentication (e.g., OAuth tokens).
- Replaced
YOUR_ACCESS_TOKEN
with a valid token to authenticate requests.
- Error Handling:
- Implemented error catching in the
fetchAppConfigs
method to handle and display errors gracefully within the component.
Step 5: Deploy and Test the Component
- Deploy the LWC:
- Use Salesforce CLI or your preferred deployment method to push the
appConfigTable
component to your Salesforce org.
- Add the Component to a Lightning Page:
- Navigate to Lightning App Builder.
- Edit or create a new Lightning page.
- Drag and drop the
appConfigTable
component onto the page. - Save and activate the page.
- Verify Functionality:
- Navigate to the page where you added the component.
- Ensure the table displays the Custom Metadata records fetched via GraphQL.
- Test error scenarios by providing incorrect GraphQL endpoint URLs or invalid access tokens to see error handling in action.
Conclusion
Querying Custom Metadata with GraphQL in LWC combines the configurability of Custom Metadata with the efficiency of GraphQL, resulting in powerful and maintainable Salesforce applications. By following the steps and best practices outlined above, Salesforce professionals can leverage this integration to build dynamic and responsive user interfaces.